Python现在很火,老季最近也想学习一下。下面我们写了一段练练手,大部分代码是从网上复制黏贴来的。
目的
采集老季博客的列表,前24页,然后将爬到的标题及单页网址打印出来。我们将会使用:
queue
列队threading
创建多线程采集BeautifulSoup
来分析网页
代码实例
#!/usr/bin/env python
# coding: utf-8
#队列自己带锁,自己阻塞,线程安全的,不用自己显式的去编写
import threading
import requests
import urllib
import queue
from bs4 import BeautifulSoup
import time
class Foo(threading.Thread):
def __init__(self, queue):
threading.Thread.__init__(self)
self.queue = queue
print('\r\nStarting Thread...',item,'\r\n')
def run(self):
while True:
key = self.queue.get()#取key列表里面的元素
print('\r\nGetting Page...',key,'\r\n')
res = self.grab(key)#运行函数grab,传的参数是key
self.queue.task_done()#取不到元素的时候自动退出程序
print(res,'\r\n')
def grab(self,key):
url = 'https://www.jiloc.com'+'/page/'+str(key)#将数字幻化成字符串
res = requests.get(url=url)
res.encoding='utf-8'
soup = BeautifulSoup(res.text,"html.parser")
d = []
for link in soup.select("h2.entry-title a"):
d.append({'title': link.get_text(), 'href':link.get("href")})
return d
if __name__ == '__main__':
time_start=time.time()
queue = queue.Queue()
key = 24
for i in range(key):
queue.put(i+1)#将key加入队列里面,用于run()的调用
for item in range(6):
t = Foo(queue)
t.setDaemon(True)#守护进程,如果主线程结束,则会退出
t.start()
queue.join()#阻塞,函数grab(1)执行完成,才会继续进行下一次grab(2)
time_end=time.time()
print('\r\ntime cost : ',round(time_end - time_start, 2),'s')
运行结果
{‘title’: ‘Make没有安装,错误sh: make: not found’, ‘href’: ‘https://www.jiloc.com/46018.html’}
{‘title’: ‘create table select from 和 insert into table select from区别’, ‘href’: ‘https://www.jiloc.com/46014.html’}
{‘title’: ‘Discuz官方论坛关闭发言权’, ‘href’: ‘https://www.jiloc.com/45806.html’}
{‘title’: ‘系统重启Reboot 后 Docker服务及容器自动启动设置’, ‘href’: ‘https://www.jiloc.com/46008.html’}
{‘title’: ‘PHP中如何将JSON格式字符串转换成http的url参数请求 图文教程’, ‘href’: ‘https://www.jiloc.com/45725.html’}{‘title’: ‘密码保护:WordPress 采集插件 wp-jpost 网站 8023mi.com 规则示例’, ‘href’: ‘https://www.jiloc.com/45038.html’}
{‘title’: ‘php中如何启用exec函数 宝塔面板中如何解除exec函数的禁用?’, ‘href’: ‘https://www.jiloc.com/44843.html’}
{‘title’: ‘LNMP1.6正式版已更新 已支持PHP7.3’, ‘href’: ‘https://www.jiloc.com/45780.html’}
{‘title’: ‘WordPress使用新浪图床后图片无法打开,如何将图片迁移到本地完整过程 图文教程’, ‘href’: ‘https://www.jiloc.com/45569.html’}{‘title’: ‘SVN 命令行使用总结’, ‘href’: ‘https://www.jiloc.com/46003.html’}
{‘title’: ‘Linux Windows MacOS 等系统中hosts的原理及作用’, ‘href’: ‘https://www.jiloc.com/44945.html’}{‘title’: ‘Vultr 在Linux系统中Nginx环境下如何建立基于域名的虚拟主机?’, ‘href’: ‘https://www.jiloc.com/45034.html’}
{‘title’: ‘基于PHP HTML5的网站测速源码’, ‘href’: ‘https://www.jiloc.com/45103.html’}
{‘title’: ‘在DEBIAN 9 或 UBUNTU 16.10 系统中配置LAMP环境’, ‘href’: ‘https://www.jiloc.com/45109.html’}
{‘title’: ‘PHP常用的文件操作函数整理’, ‘href’: ‘https://www.jiloc.com/45084.html’}
{‘title’: ‘Nginx常用屏蔽规则,让网站更安全’, ‘href’: ‘https://www.jiloc.com/45087.html’}
{‘title’: ‘CN2 GT(GIS)和CN2 GIA的区别办法’, ‘href’: ‘https://www.jiloc.com/45092.html’}
{‘title’: ‘php数组去除空值array_filter函数使用方法 代码实例’, ‘href’: ‘https://www.jiloc.com/45956.html’}{‘title’: ‘ThinkPHP 在Apache和Nginx中伪静态规则如何写?’, ‘href’: ‘https://www.jiloc.com/45030.html’}
{‘title’: ‘Windows 10启用Linux子系统(WSL) 图文教程’, ‘href’: ‘https://www.jiloc.com/45016.html’}
{‘title’: ‘LNMP一键安装包(Linux+Nginx+Mysql/MariaDB+PHP)图文教程’, ‘href’: ‘https://www.jiloc.com/45782.html’}
{‘title’: ‘Vultr 优惠活动 注册即送$50美金,16个机房可选,按时计费’, ‘href’: ‘https://www.jiloc.com/45539.html’}
{‘title’: ‘ECharts图表点击柱状打开a链接’, ‘href’: ‘https://www.jiloc.com/45721.html’}
{‘title’: ‘解决WordPress全站开启https后的此网页包含过多的重定向问题’, ‘href’: ‘https://www.jiloc.com/45079.html’}
{‘title’: ‘WordPress正确引入JS和CSS文件方法’, ‘href’: ‘https://www.jiloc.com/44838.html’}
{‘title’: ‘[Vultr] VPS 怎么给账户充值余额?’, ‘href’: ‘https://www.jiloc.com/44727.html’}
{‘title’: ‘搬瓦工美国cn2 vps服务器推荐购买方案 支持支付宝/微信’, ‘href’: ‘https://www.jiloc.com/45777.html’}
{‘title’: ‘腾讯云如何更换服务器新的IP’, ‘href’: ‘https://www.jiloc.com/45770.html’}
{‘title’: ‘ECharts图表中XY轴数据过多导致重叠显示不全问题如何解决 图文教程’, ‘href’: ‘https://www.jiloc.com/45714.html’}
{‘title’: ‘Linux如何使用UnixBench脚本测试系统服务器性能跑分 图文教程’, ‘href’: ‘https://www.jiloc.com/45707.html’}
{‘title’: ‘WordPress中如何引入默认jQuery并在特别jQuery版本中使用$()写法’, ‘href’: ‘https://www.jiloc.com/44834.html’}
{‘title’: ‘[Vultr]怎么续费?怎么取消自动续费?’, ‘href’: ‘https://www.jiloc.com/44724.html’}{‘title’: ‘如何利用Shell脚本程序将WordPress网站中的新浪图片批量下载到服务器本地’, ‘href’: ‘https://www.jiloc.com/45575.html’}
{‘title’: ‘docker logs-查看docker容器日志’, ‘href’: ‘https://www.jiloc.com/46207.html’}
{‘title’: ‘PHP7 开启 Zend Opcache 加速网站服务器 图文教程’, ‘href’: ‘https://www.jiloc.com/46199.html’}
{‘title’: ‘jQuery获取DropDownList下拉框操作Javascript代码实例 代码总结’, ‘href’: ‘https://www.jiloc.com/46195.html’}
{‘title’: ‘Debian9系统如何修改SSH端口 图文教程’, ‘href’: ‘https://www.jiloc.com/45643.html’}
{‘title’: ‘Debian9 Stretch如何更换apt源’, ‘href’: ‘https://www.jiloc.com/45645.html’}
{‘title’: ‘Visual Studio Code 终端中使用 SVN not found’, ‘href’: ‘https://www.jiloc.com/45996.html’}{‘title’: ‘新浪博客宣布“相册”功能下线:8月停止导出’, ‘href’: ‘https://www.jiloc.com/45564.html’}
{‘title’: ‘全能下载工具Motrix,支持BT、磁力链、百度网盘等资源’, ‘href’: ‘https://www.jiloc.com/45560.html’}
{‘title’: ‘WordPress 开源采集插件 WP-JPost V0.8 ChangeLog’, ‘href’: ‘https://www.jiloc.com/45076.html’}
{‘title’: ‘[腾讯云]四月每天三场秒杀优惠活动 云服务器/数据库/短信’, ‘href’: ‘https://www.jiloc.com/45067.html’}
{‘title’: ‘WordPress 函数 :get_post_statuses 检索所有文章帖子状态值’, ‘href’: ‘https://www.jiloc.com/44831.html’}
{‘title’: ‘[Vultr]VPS 申请退款销号图文教程’, ‘href’: ‘https://www.jiloc.com/44720.html’}
{‘title’: ‘如何使用SecureCRT实现命令行的上传下载文件(使用sz与rz命令)图文教程’, ‘href’: ‘https://www.jiloc.com/45756.html’}
{‘title’: ‘新浪图床近期不稳定论自建图床的重要性’, ‘href’: ‘https://www.jiloc.com/45556.html’}
{‘title’: ‘jQuery操作radio单选框Javascript代码实例 图文教程’, ‘href’: ‘https://www.jiloc.com/46192.html’}
{‘title’: ‘php生成GD图片不显示问题 解决办法 图文教程’, ‘href’: ‘https://www.jiloc.com/46189.html’}
{‘title’: ‘php下安装配置mbstring模块’, ‘href’: ‘https://www.jiloc.com/44824.html’}
{‘title’: ‘[Vultr]如何删除VPS/暂停VPS 图文教程’, ‘href’: ‘https://www.jiloc.com/44716.html’}
{‘title’: ‘[Vultr]VPS如何查看账单?怎么查看Vultr用了多少钱?’, ‘href’: ‘https://www.jiloc.com/44708.html’}{‘title’: ‘Linux系统中使用PHP脚本定时监控交换机端口流量并添加删除修改交换机规则控制流量’, ‘href’: ‘https://www.jiloc.com/45749.html’}
{‘title’: ‘阿里云云数据库RDS 首次用户可享半年10元优惠 云数据库MySQL首次购买专享福利’, ‘href’: ‘https://www.jiloc.com/45549.html’}{‘title’: ‘Vultr中Nginx环境下PHP安全设置 图文教程’, ‘href’: ‘https://www.jiloc.com/45010.html’}
{‘title’: ‘pip安装时ReadTimeoutError解决办法’, ‘href’: ‘https://www.jiloc.com/46183.html’}
{‘title’: ‘PHP代码检测web环境是否开启mod_rewrite模块’, ‘href’: ‘https://www.jiloc.com/44819.html’}
{‘title’: ‘php程序中代码前面加上@符号是什么意思?’, ‘href’: ‘https://www.jiloc.com/44816.html’}
{‘title’: ‘Docker安装Mariadb数据库 设置root密码 图文教程’, ‘href’: ‘https://www.jiloc.com/45880.html’}
{‘title’: ‘Ubuntu系统下BT宝塔面板新增快捷命令行处理 修改端口/解除域名绑定/重启重置密码等’, ‘href’: ‘https://www.jiloc.com/45062.html’}
{‘title’: ‘Git如何设置两个远程仓库地址?’, ‘href’: ‘https://www.jiloc.com/45637.html’}
{‘title’: ‘RamNode 云充值优惠 $5美金起充 新老客户都可以参与 额外赠送50%额度’, ‘href’: ‘https://www.jiloc.com/46180.html’}
{‘title’: ‘WordPress 下载主题模板、更新报错 No working transports found解决办法’, ‘href’: ‘https://www.jiloc.com/44812.html’}{‘title’: ‘PHP开发中跳出多层循环方法’, ‘href’: ‘https://www.jiloc.com/45746.html’}
{‘title’: ‘Linode新上线加拿大多伦多Toronto, ON数据中心 注册即送10美元 2019 Linode优惠码 1核1G月付5美元起 按小时计费’, ‘href’: ‘https://www.jiloc.com/45545.html’}
{‘title’: ‘WordPress安装环境需要PHP与MySQL版本要求’, ‘href’: ‘https://www.jiloc.com/45057.html’}
{‘title’: ‘记一次PHP MySQL注入入侵’, ‘href’: ‘https://www.jiloc.com/45989.html’}{‘title’: ‘Vultr VPS 下 Windows 系统 服务器 中配置XAMPP环境搭建 WordPress博客图文教程’, ‘href’: ‘https://www.jiloc.com/44916.html’}
{‘title’: ‘WordPress插件开发 register_post_type 设定菜单 位置 结合 add_submenu_page 添加自定义设置页面’, ‘href’: ‘https://www.jiloc.com/45949.html’}
{‘title’: ‘Docker 安装 PHP 并配合 Nginx 运行 phpinfo’, ‘href’: ‘https://www.jiloc.com/45874.html’}
{‘title’: ‘[Vultr]Linux系统中iptables防火墙 常用规则整理’, ‘href’: ‘https://www.jiloc.com/45006.html’}{‘title’: ‘Vultr 优惠活动 注册即送$50美金,16个机房可选,按时计费’, ‘href’: ‘https://www.jiloc.com/45539.html’}
{‘title’: ‘Linux下使用Oneinstack Web环境一键升级到最新版本 图文教程’, ‘href’: ‘https://www.jiloc.com/45049.html’}
{‘title’: ‘RamNode新品OpenStack云服务器平台开通及主机方案 图文教程’, ‘href’: ‘https://www.jiloc.com/46161.html’}
{‘title’: ‘[Vultr]VPS 流量用完了怎么办?’, ‘href’: ‘https://www.jiloc.com/44704.html’}{‘title’: ‘WordPress 插件开发 如何一次性输入多个标签Tags搜索文章内容?’, ‘href’: ‘https://www.jiloc.com/45944.html’}
{‘title’: ‘PHP如何登录连接TELNET并执行交换机命令类 图文教程’, ‘href’: ‘https://www.jiloc.com/45742.html’}
{‘title’: ‘腾讯云 数字生态 – 每天五场秒杀 爆款1C1G云服务器首年99元/香港服务器199元’, ‘href’: ‘https://www.jiloc.com/45629.html’}
{‘title’: ‘零基础如何在Linux系统下搭建WordPress?’, ‘href’: ‘https://www.jiloc.com/45041.html’}{‘title’: ‘Vultr VPS 如何利用phpmyadmin建立数据库?’, ‘href’: ‘https://www.jiloc.com/44927.html’}
{‘title’: ‘WordPress中安装插件需要ftp怎么办?’, ‘href’: ‘https://www.jiloc.com/44809.html’}
{‘title’: ‘Docker 安装 Nginx 映射本地文件 多虚拟主机 图文教程’, ‘href’: ‘https://www.jiloc.com/45860.html’}{‘title’: ‘Vultr VPS 安装了Debian系统后如何修改iptables规则配置 图文教程’, ‘href’: ‘https://www.jiloc.com/45736.html’}{‘title’: ‘[Enoctus] VPS 月付$10/无限流量/1GB内存/24GB SSD 硬盘’, ‘href’: ‘https://www.jiloc.com/44997.html’}{‘title’: ‘搬瓦工问答整理’, ‘href’: ‘https://www.jiloc.com/45165.html’}
{‘title’: ‘腾讯云限时秒杀活动 – 仅限新人云服务器低至198元一年’, ‘href’: ‘https://www.jiloc.com/45981.html’}
{‘title’: ‘搬瓦工VPS 补货DC9 CN2 GIA限量年付$39.99美金套餐’, ‘href’: ‘https://www.jiloc.com/46158.html’}{‘title’: ‘Vultr VPS 中 如何利用phpMyAdmin修改数据库密码?’, ‘href’: ‘https://www.jiloc.com/44923.html’}{‘title’: ‘[Vultr]发工单联系客服 图文教程’, ‘href’: ‘https://www.jiloc.com/44697.html’}
{‘title’: ‘[Vultr]如何删除服务器 停止计费’, ‘href’: ‘https://www.jiloc.com/44693.html’}
{‘title’: ‘MacOS系统中Mojave如何设置简单简短密码 解除密码最小长度4位’, ‘href’: ‘https://www.jiloc.com/45732.html’}
{‘title’: ‘ECharts图表中的XY轴长度过大如何截取?’, ‘href’: ‘https://www.jiloc.com/45728.html’}
{‘title’: ‘PHP 检索整个数据库 所有表 所有字段 包含某个关键字’, ‘href’: ‘https://www.jiloc.com/45939.html’}
{‘title’: ‘Docker中快速删除所有容器’, ‘href’: ‘https://www.jiloc.com/45868.html’}
{‘title’: ‘[Vultr]VPS支付方式 – AliPay支付宝/WeChat微信/Cred Card信用卡/PayPal贝宝等 以及付款区别’, ‘href’: ‘https://www.jiloc.com/45152.html’}
{‘title’: ‘插件实现WordPress上传图片单独存到至腾讯云COS对象存储’, ‘href’: ‘https://www.jiloc.com/45135.html’}
{‘title’: ‘[WordPress] 批量删除所有文章的特色图片 函数代码’, ‘href’: ‘https://www.jiloc.com/44912.html’}
{‘title’: ‘WordPress 插件开发 如何新建自定义页面?’, ‘href’: ‘https://www.jiloc.com/45934.html’}{‘title’: ‘[Vultr]是怎么收费的?Vultr计时收费模式详解’, ‘href’: ‘https://www.jiloc.com/44682.html’}
{‘title’: ‘Windows VPS服务器快速修改默认3389端口提高安全性能’, ‘href’: ‘https://www.jiloc.com/44988.html’}
{‘title’: ‘[Vutlr]Linux Cetnos Debian 使用putty登陆 vultr VPS 服务器’, ‘href’: ‘https://www.jiloc.com/44661.html’}{‘title’: ‘PHP保留两位小数 四舍五入 代码实例’, ‘href’: ‘https://www.jiloc.com/46153.html’}{‘title’: ‘MacOS XAMPP 目录权限设置 图文教程’, ‘href’: ‘https://www.jiloc.com/44481.html’}
{‘title’: ‘WordPress安装时出现”查询SELECT wp_时,WordPress数据库发生Unknown column ‘wp_’ in ‘field list’错误”’, ‘href’: ‘https://www.jiloc.com/44792.html’}
{‘title’: ‘[Vultr]创建新的VPS服务器 图文教程’, ‘href’: ‘https://www.jiloc.com/44675.html’}
{‘title’: ‘Windows 系统中如何进入注册表?’, ‘href’: ‘https://www.jiloc.com/44992.html’}
{‘title’: ‘利用 腾讯云开发者平台 Cloud Studio 免费空间 一键部署 WordPress 可绑定自定义域名 支持https’, ‘href’: ‘https://www.jiloc.com/45113.html’}
{‘title’: ‘php中file_get_contents 和 curl 区别’, ‘href’: ‘https://www.jiloc.com/44909.html’}
{‘title’: ‘WordPress插件开发 在注册自定义文章后如何修改文章默认固定链接地址?’, ‘href’: ‘https://www.jiloc.com/45929.html’}{‘title’: ‘jQuery获取append添加html内容后的动态元素:live()和on()’, ‘href’: ‘https://www.jiloc.com/45857.html’}{‘title’: ‘阿里云Hi拼购活动 – 阿里云服务器优惠团购低至年付¥199元/老用户年付¥248元’, ‘href’: ‘https://www.jiloc.com/45619.html’}{‘title’: ‘Windows下如何查看系统版本位数 32|64位系统’, ‘href’:
‘https://www.jiloc.com/44668.html’}
{‘title’: ‘宝塔面板安装不同PHP版本 切换WordPress PHP版本 图文教程’, ‘href’: ‘https://www.jiloc.com/44973.html’}{‘title’: ‘php代码中检测当前版本及环境是否支持某个函数’, ‘href’: ‘https://www.jiloc.com/44655.html’}
{‘title’: ‘MacOS 连接 Windows远程桌面 Microsoft Remote Desktop for Mac 下载 图文教程’, ‘href’: ‘https://www.jiloc.com/44475.html’}
{‘title’: ‘[WordPress]如何使用百度代码推送文章 百度快速收录 图文教程’, ‘href’: ‘https://www.jiloc.com/44904.html’}
{‘title’: ‘[搬瓦工]新上线DC6机房CN2 GIA限量版/1核512MB内存/1Gbps带宽/500G流量/KVM架构/46.87美元/年’, ‘href’: ‘https://www.jiloc.com/44887.html’}
{‘title’: ‘WordPress 如何获取文章发表用户ID’, ‘href’: ‘https://www.jiloc.com/45976.html’}{‘title’: ‘[Vutlr]Linux Cetnos Debian 使用putty登陆 vultr VPS 服务器’, ‘href’: ‘https://www.jiloc.com/44661.html’}
{‘title’: ‘Vue.js 如何设置Select Option下拉值’, ‘href’: ‘https://www.jiloc.com/46147.html’}{‘title’: ‘[Ramnode]仅需$3每月/512M 内存/10G SSD/1T 流量/1Gbps/KVM/洛杉矶/西雅图’, ‘href’: ‘https://www.jiloc.com/44609.html’}{‘title’: ‘Windows Server 安装配置IIS+MySQL+PHP环境的详细图文教程–MySQL篇’, ‘href’: ‘https://www.jiloc.com/44772.html’}{‘title’: ‘MAC OS下切换默认终端为zsh 图文教程’, ‘href’: ‘https://www.jiloc.com/44472.html’}
{‘title’: ‘网站恶意镜像 原理和Javascript解决方案’, ‘href’: ‘https://www.jiloc.com/44884.html’}
{‘title’: ‘PHP 基于Redis/Memcached的高并发秒杀 锁 设计思路 代码示例’, ‘href’: ‘https://www.jiloc.com/44364.html’}
{‘title’: ‘Vultr VPS 宝塔面板中 安装WordPress后更改“固定链接”后 页面404解决方法 图文教程’, ‘href’: ‘https://www.jiloc.com/44965.html’}
{‘title’: ‘MacOS homebrew 加速设置 图文教程’, ‘href’: ‘https://www.jiloc.com/44469.html’}
{‘title’: ‘腾讯云秒杀活动仅限新人 香港云服务器云服务器1C1G年付¥452元’, ‘href’: ‘https://www.jiloc.com/45614.html’}
{‘title’: ‘BING、百度、有道平台免费翻译API’, ‘href’: ‘https://www.jiloc.com/44876.html’}
{‘title’: ‘密码保护:WordPress 自动采集插件 wp-jpost 采集 网站 fanjian.net 规则示例’, ‘href’: ‘https://www.jiloc.com/44359.html’}
{‘title’: ‘Vultr VPS服务器 XAMPP 在Apache下如何添加自定义虚拟主机绑定多个域名?’, ‘href’: ‘https://www.jiloc.com/44937.html’}{‘title’: ‘[Vultr]$10/月/2G 内存/55G SSD/2T 流量/KVM/日本/新加坡/洛杉矶等15机房 支持支付
宝’, ‘href’: ‘https://www.jiloc.com/44601.html’}
{‘title’: ‘PHP 开发 foreach list 配套 代码实例’, ‘href’: ‘https://www.jiloc.com/45926.html’}
{‘title’: ‘搬瓦工bandwagonhost VPS忘记续费VPS被Suspended 怎么办?’, ‘href’: ‘https://www.jiloc.com/44415.html’}
{‘title’: ‘CVE-2019-0708 Remote Desktop Services Remote Code Execution Vulnerability 微软远程桌面漏洞’, ‘href’: ‘https://www.jiloc.com/45611.html’}
{‘title’: ‘WordPress 开源采集插件 WP-JPost V0.7.7 ChangeLog’, ‘href’: ‘https://www.jiloc.com/44864.html’}
{‘title’: ‘Vultr新增Object Storage对象存储’, ‘href’: ‘https://www.jiloc.com/46141.html’}
{‘title’: ‘Windows Server 安装配置IIS+MySQL+PHP环境的详细图文教程–PHP篇’, ‘href’: ‘https://www.jiloc.com/44750.html’}{‘title’: ‘[Buyvm]$2/月/512MB内存/10GB SSD空间/不限流量/KVM/拉斯维加斯CN2 GIA 可使用支付宝’, ‘href’: ‘https://www.jiloc.com/44557.html’}{‘title’: ‘微信小程序开发 批量选择上传图片 代码实例’, ‘href’: ‘https://www.jiloc.com/45853.html’}
{‘title’: ‘Linux内核TCP SACK Panic远程拒绝服务漏洞’, ‘href’: ‘https://www.jiloc.com/45972.html’}
{‘title’: ‘老季博客系统Debian9 Stretch及环境php7.3升级完成’, ‘href’: ‘https://www.jiloc.com/45596.html’}
{‘title’: ‘php中如何启用exec函数 宝塔面板中如何解除exec函数的禁用?’, ‘href’: ‘https://www.jiloc.com/44843.html’}
{‘title’: ‘Docker 本地基本搭建Nginx+PHP-FPM+MARIADB 命令’, ‘href’: ‘https://www.jiloc.com/45920.html’}
{‘title’: ‘WordPress 开源采集插件 WP-JPost V0.6.2 ChangeLog’, ‘href’: ‘https://www.jiloc.com/44407.html’}
{‘title’: ‘密码保护:WordPress 自动采集插件 wp-jpost 采集 网站 weijj.com 规则示例’, ‘href’: ‘https://www.jiloc.com/44355.html’}
{‘title’: ‘Google Compute Engine GCE 免费300美金/一年使用期限 注册使用 图文教程’, ‘href’: ‘https://www.jiloc.com/44278.html’}{‘title’: ‘[RamNode]稳定建站之选 已接入支付宝、微信支付’, ‘href’: ‘https://www.jiloc.com/44551.html’}{‘title’: ‘Linux TCP “SACK PANIC” 远程拒绝服务漏洞 处理应对方案 图文教程’, ‘href’: ‘https://www.jiloc.com/45849.html’}
{‘title’: ‘MacOS 更新 MOJAVE 10.14 星际争霸2 进入游戏鼠标无法移动 解决方案’, ‘href’: ‘https://www.jiloc.com/44403.html’}
{‘title’: ‘Windows Server 2012 R2 解除文件下载限制’, ‘href’: ‘https://www.jiloc.com/44398.html’}
{‘title’: ‘PHP如何正则表达式获取SQL语句中的表名?’, ‘href’: ‘https://www.jiloc.com/45914.html’}
{‘title’: ‘WordPress 插件设置’, ‘href’: ‘https://www.jiloc.com/44232.html’}
{‘title’: ‘WordPress 插件开发 激活插件时添加通知 代码实例’, ‘href’: ‘https://www.jiloc.com/45966.html’}
{‘title’: ‘某面板6.x版本前台存储xss+后台csrf组合拳getshell 图文教程’, ‘href’: ‘https://www.jiloc.com/44342.html’}
{‘title’: ‘Vultr Debian 8 x64环境下一键安装Windows7系统’, ‘href’: ‘https://www.jiloc.com/44395.html’}{‘title’: ‘香港VPS/1核/1G/25G SSD/1T流量/30M带宽/年付144元’, ‘href’: ‘https://www.jiloc.com/44548.html’}{‘title’: ‘解决Chrome插件安装时出现 程序包无效:”CRX_HEADER_INVALID”。’, ‘href’: ‘https://www.jiloc.com/45839.html’}
{‘title’: ‘WordPress 插入媒体’, ‘href’: ‘https://www.jiloc.com/44162.html’}{‘title’: ‘WordPress 添加评论’, ‘href’: ‘https://www.jiloc.com/44094.html’}{‘title’: ‘WordPress 添加类别’, ‘href’: ‘https://www.jiloc.com/44215.html’}
{‘title’: ‘WordPress 编辑类别’, ‘href’: ‘https://www.jiloc.com/44211.html’}
{‘title’: ‘WordPress 主题管理’, ‘href’: ‘https://www.jiloc.com/44043.html’}
{‘title’: ‘WordPress 插件开发 自定义文章类型中 add_meta_box 如何使用原生的分类/标签显示并保存数据?’, ‘href’: ‘https://www.jiloc.com/45909.html’}
{‘title’: ‘php数组去除空值array_filter函数使用方法 代码实例’, ‘href’: ‘https://www.jiloc.com/45956.html’}
{‘title’: ‘WordPress插件开发 register_post_type 设定菜单 位置 结合 add_submenu_page 添加自定义设置页面’, ‘href’: ‘https://www.jiloc.com/45949.html’}
{‘title’: ‘阿里云轻量应用服务器 一键脚本 DD Windows 2008 图文教程’, ‘href’: ‘https://www.jiloc.com/44541.html’}
{‘title’: ‘Javascript开发jQuery Ajax 返回值如何阻止form表单提交?’, ‘href’: ‘https://www.jiloc.com/45905.html’}
{‘title’: ‘WordPress 编辑媒体’, ‘href’: ‘https://www.jiloc.com/44157.html’}
{‘title’: ‘#活动# 腾讯云 – 2核8G(上海区)1折 / 2核4G(上海区)2折 / 1核1G(上海区)3折’, ‘href’: ‘https://www.jiloc.com/44274.html’}
{‘title’: ‘密码保护:WordPress 自动采集插件 wp-jpost 采集 网站 dytt8.net 规则示例’, ‘href’: ‘https://www.jiloc.com/44380.html’}
{‘title’: ‘PHP实用基础如何实现删除数组中的特定元素 代码实例’, ‘href’: ‘https://www.jiloc.com/45830.html’}{‘title’: ‘搬瓦工如何选择登录已购VPS方案面板?’, ‘href’: ‘https://www.jiloc.com/44522.html’}{‘title’: ‘WordPress 插件 Redis Object Cache 搭配 WP Super Cache 同一服务器多站点 缓存配置 防止串内容’, ‘href’: ‘https://www.jiloc.com/43916.html’}{‘title’: ‘什么是Docker?Docker的架构是什么样的?’, ‘href’: ‘https://www.jiloc.com/45890.html’}{‘title’: ‘WordPress 编辑评论’, ‘href’: ‘https://www.jiloc.com/44088.html’}
{‘title’: ‘密码保护:WordPress 自动采集插件 wp-jpost 采集 网站 jiajusmart.com 规则示例’, ‘href’: ‘https://www.jiloc.com/44339.html’}{‘title’: ‘WordPress 删除类别’, ‘href’: ‘https://www.jiloc.com/44205.html’}{‘title’: ‘WordPress 开发 wp_category_checklist 将分类显示为checkbox格式’, ‘href’: ‘https://www.jiloc.com/44269.html’}
{‘title’: ‘PHP 基于Redis/Memcached的高并发秒杀 锁 设计思路 代码示例’, ‘href’: ‘https://www.jiloc.com/44364.html’}
{‘title’: ‘WordPress 添加页面’, ‘href’: ‘https://www.jiloc.com/44152.html’}{‘title’: ‘WordPress 审核评论’, ‘href’: ‘https://www.jiloc.com/44084.html’}
{‘title’: ‘WordPress函数:register post type (自定义文章类型)用法和范例’, ‘href’: ‘https://www.jiloc.com/43831.html’}
{‘title’: ‘WordPress 开发 wp_dropdown_categories 下拉式框显示所分类目录标签函数’, ‘href’: ‘https://www.jiloc.com/44267.html’}
{‘title’: ‘密码保护:WordPress 自动采集插件 wp-jpost 采集 网站 fanjian.net 规则示例’, ‘href’: ‘https://www.jiloc.com/44359.html’}{‘title’: ‘搬瓦工VPS(BandwagonHost)自主任意更换机房实现换IP’, ‘href’: ‘https://www.jiloc.com/44516.html’}
{‘title’: ‘WordPress 发布页面’, ‘href’: ‘https://www.jiloc.com/44141.html’}
{‘title’: ‘WordPress 分类安排’, ‘href’: ‘https://www.jiloc.com/44201.html’}{‘title’: ‘WordPress 自定义主题’, ‘href’: ‘https://www.jiloc.com/44035.html’}
{‘title’: ‘WordPress 安装’, ‘href’: ‘https://www.jiloc.com/44261.html’}
{‘title’: ‘Discuz! 经典代码 PHP加密解密函数’, ‘href’: ‘https://www.jiloc.com/43907.html’}
{‘title’: ‘PHP实现域名授权的两种方法 图文教程’, ‘href’: ‘https://www.jiloc.com/43905.html’}{‘title’: ‘WordPress 查看插件’, ‘href’: ‘https://www.jiloc.com/44081.html’}
{‘title’: ‘WordPress 免费采集插件 WP-JPost 定时自动增量采集任务 设置图文教程’, ‘href’: ‘https://www.jiloc.com/43875.html’}
{‘title’: ‘PHP数组函数–array_filter 用法详解’, ‘href’: ‘https://www.jiloc.com/45827.html’}
{‘title’: ‘搬瓦工KiwiVM面板新手易用入门教学详细图文讲解’, ‘href’: ‘https://www.jiloc.com/44508.html’}
{‘title’: ‘wordpress使用query_posts()函数WP_Query类获取指定分类中的日志 代码示例’, ‘href’: ‘https://www.jiloc.com/43848.html’}
{‘title’: ‘WordPress 编辑页面’, ‘href’: ‘https://www.jiloc.com/44137.html’}
{‘title’: ‘WordPress 添加帖子’, ‘href’: ‘https://www.jiloc.com/44197.html’}
{‘title’: ‘WordPress 函数:get_template_part()调用你的自定义模板’, ‘href’: ‘https://www.jiloc.com/43840.html’}
{‘title’: ‘Vultr更换新UI界面 新增High Frequency Compute 更换新LOGO’, ‘href’: ‘https://www.jiloc.com/45820.html’}
{‘title’: ‘上海溯飏船舶物资有限公司’, ‘href’: ‘https://www.jiloc.com/43797.html’}
{‘title’: ‘WordPress 在插件或模板开发中使用 session 图文教程’, ‘href’: ‘https://www.jiloc.com/43902.html’}{‘title’: ‘WordPress 安装插件’, ‘href’: ‘https://www.jiloc.com/44078.html’}
{‘title’: ‘WordPress 窗口管理’, ‘href’: ‘https://www.jiloc.com/44020.html’}{‘title’: ‘WordPress 编辑帖子’, ‘href’: ‘https://www.jiloc.com/44193.html’}
{‘title’: ‘Linux命令详解:crontab 定时任务’, ‘href’: ‘https://www.jiloc.com/43878.html’}
{‘title’: ‘jQuery全文检索表格 特定文字变色高亮红色 图文教程’, ‘href’: ‘https://www.jiloc.com/45810.html’}{‘title’: ‘搬瓦工VPS推荐方案’, ‘href’: ‘https://www.jiloc.com/44494.html’}
{‘title’: ‘WordPress函数:add meta box(自定义添加Meta模块)’, ‘href’: ‘https://www.jiloc.com/43846.html’}
{‘title’: ‘WordPress 删除页面’, ‘href’: ‘https://www.jiloc.com/44131.html’}{‘title’: ‘WordPress 插件定制’, ‘href’: ‘https://www.jiloc.com/44073.html’}
{‘title’: ‘WordPress 仪表盘’, ‘href’: ‘https://www.jiloc.com/44252.html’}
{‘title’: ‘密码保护:WordPress 自动采集插件 wp-jpost 采集 网站 chinaznj.com 规则示例’, ‘href’: ‘https://www.jiloc.com/44336.html’}
{‘title’: ‘WordPress Robots.txt 写法优化 示例’, ‘href’: ‘https://www.jiloc.com/43795.html’}
{‘title’: ‘WordPress 开发必了解 过滤器(Filters):apply_filters和add_filter 用法和实例’, ‘href’: ‘https://www.jiloc.com/43829.html’}
{‘title’: ‘WordPress 背景’, ‘href’: ‘https://www.jiloc.com/44014.html’}
{‘title’: ‘WordPress 函数:add_theme_support()让你的主题支持特定的功能’, ‘href’: ‘https://www.jiloc.com/43838.html’}
{‘title’: ‘密码保护:WordPress 自动采集插件 wp-jpost 采集 网站 webhtm.cn 规则示例’, ‘href’: ‘https://www.jiloc.com/44331.html’}
{‘title’: ‘WordPress 主题制作基本模版文件以及基本函数 详解’, ‘href’: ‘https://www.jiloc.com/43793.html’}
{‘title’: ‘WordPress 添加标签’, ‘href’: ‘https://www.jiloc.com/44126.html’}
{‘title’: ‘WordPress 删除帖子’, ‘href’: ‘https://www.jiloc.com/44187.html’}
{‘title’: ‘WordPress 通用设置’, ‘href’: ‘https://www.jiloc.com/44250.html’}
{‘title’: ‘WordPress 开源采集插件 WP-JPost V0.6.1 ChangeLog’, ‘href’: ‘https://www.jiloc.com/44322.html’}
{‘title’: ‘百度翻译 申请API Key 图文教程’, ‘href’: ‘https://www.jiloc.com/43783.html’}{‘title’: ‘WordPress 用户角色’, ‘href’: ‘https://www.jiloc.com/44066.html’}{‘title’: ‘WordPress 编辑标签’, ‘href’: ‘https://www.jiloc.com/44122.html’}
{‘title’: ‘WordPress 预览帖子’, ‘href’: ‘https://www.jiloc.com/44183.html’}
{‘title’: ‘WordPress 写作设置’, ‘href’: ‘https://www.jiloc.com/44247.html’}
{‘title’: ‘PC/WAP 常见USER-AGENT值 列表’, ‘href’: ‘https://www.jiloc.com/44317.html’}
{‘title’: ‘WordPress 主机转移’, ‘href’: ‘https://www.jiloc.com/44005.html’}{‘title’: ‘WordPress 删除标签’, ‘href’: ‘https://www.jiloc.com/44116.html’}
{‘title’: ‘WordPress 自动采集插件 wp-jpost 采集网站 hecaijing.com 规则示例’, ‘href’: ‘https://www.jiloc.com/43872.html’}
{‘title’: ‘WordPress 阅读设置’, ‘href’: ‘https://www.jiloc.com/44244.html’}{‘title’: ‘理解WordPress模板开发中无处不在的主循环’, ‘href’: ‘https://www.jiloc.com/43827.html’}
{‘title’: ‘国内便宜云主机 七牛云 1元/月(仅限1个月)/ 需实名 国内多机房可选’, ‘href’: ‘https://www.jiloc.com/43774.html’}
{‘title’: ‘WordPress 发布帖子’, ‘href’: ‘https://www.jiloc.com/44178.html’}
{‘title’: ‘WordPress MYSQL 数据库及各表结构’, ‘href’: ‘https://www.jiloc.com/43823.html’}
{‘title’: ‘WordPress判断文章分类函数in_category和is_category区别’, ‘href’: ‘https://www.jiloc.com/43870.html’}{‘title’: ‘WordPress 函数do_action()详解 应用实例’, ‘href’: ‘https://www.jiloc.com/43825.html’}
{‘title’: ‘小内存VPS Caddy+php 配置 图文教程’, ‘href’: ‘https://www.jiloc.com/43768.html’}
{‘title’: ‘WordPress 添加链接’, ‘href’: ‘https://www.jiloc.com/44112.html’}
{‘title’: ‘WordPress 媒体库’, ‘href’: ‘https://www.jiloc.com/44174.html’}
{‘title’: ‘WordPress分类列表函数:wp_list_categories用法及参数详解举例’, ‘href’: ‘https://www.jiloc.com/43867.html’}
{‘title’: ‘WordPress 版本更新’, ‘href’: ‘https://www.jiloc.com/43993.html’}
{‘title’: ‘MacOS 下修改 iTunes 备份文件路径 图文教程’, ‘href’: ‘https://www.jiloc.com/43900.html’}
{‘title’: ‘WordPress 开源采集插件 WP-JPost V0.6.0 ChangeLog’, ‘href’: ‘https://www.jiloc.com/44314.html’}{‘title’: ‘WordPress 讨论设置’, ‘href’: ‘https://www.jiloc.com/44241.html’}
{‘title’: ‘WordPress 函数 wp_list_comments()使用回调函数自定义评论展示方式’, ‘href’: ‘https://www.jiloc.com/43821.html’}
{‘title’: ‘新浪免费图床 微博相册 使用方法 图文教程’, ‘href’: ‘https://www.jiloc.com/43766.html’}{‘title’: ‘WordPress 垃圾邮件防护’, ‘href’: ‘https://www.jiloc.com/43989.html’}
{‘title’: ‘WordPress 备份和恢复’, ‘href’: ‘https://www.jiloc.com/43979.html’}
{‘title’: ‘WordPress 添加用户’, ‘href’: ‘https://www.jiloc.com/44065.html’}
{‘title’: ‘wordpress函数:get_permalink()获取文章页面的固定链接’, ‘href’: ‘https://www.jiloc.com/43865.html’}
{‘title’: ‘WordPress 编辑链接’, ‘href’: ‘https://www.jiloc.com/44105.html’}{‘title’: ‘BT宝塔面板 磁盘爆满处理方法 图文教程’, ‘href’: ‘https://www.jiloc.com/43896.html’}{‘title’: ‘WordPress 添加媒体’, ‘href’: ‘https://www.jiloc.com/44167.html’}
{‘title’: ‘WordPress 自动采集插件 wp-jpost 采集 Discuz论坛 网站 hostloc.com 规则示例’, ‘href’: ‘https://www.jiloc.com/44312.html’}
{‘title’: ‘WordPress 用户照片’, ‘href’: ‘https://www.jiloc.com/44061.html’}{‘title’: ‘WordPress 函数:register_sidebar()创建主题侧边栏’, ‘href’: ‘https://www.jiloc.com/43819.html’}{‘title’: ‘WordPress函数:wp_get_archives根据日期显示日志归档详解举例’, ‘href’: ‘https://www.jiloc.com/43863.html’}
{‘title’: ‘WordPress 优化’, ‘href’: ‘https://www.jiloc.com/43963.html’}{‘title’: ‘WordPress 媒体设置’, ‘href’: ‘https://www.jiloc.com/44238.html’}
{‘title’: ‘WordPress 主题插件的多语言工具poedit包最新汉化版使用 图文教程’, ‘href’: ‘https://www.jiloc.com/43811.html’}
{‘title’: ‘WordPress 删除链接’, ‘href’: ‘https://www.jiloc.com/44099.html’}{‘title’: ‘WordPress 插件开发 支持多语言 国际化 图文教程’, ‘href’: ‘https://www.jiloc.com/43890.html’}
{‘title’: ‘WordPress函数:load_plugin_textdomain 插件多语言 国际化 本地化’, ‘href’: ‘https://www.jiloc.com/43892.html’}{‘title’: ‘WordPress 固定链接设置’, ‘href’: ‘https://www.jiloc.com/44235.html’}{‘title’: ‘WordPress 编辑用户’, ‘href’: ‘https://www.jiloc.com/44057.html’}
{‘title’: ‘wordpress使用register_post_type 函数创建自定义文章类型’, ‘href’: ‘https://www.jiloc.com/43851.html’}{‘title’: ‘WordPress配置核心文件wp-config.php详解’, ‘href’: ‘https://www.jiloc.com/43809.html’}
{‘title’: ‘MacOS升级后出现的 xcrun: error: invalid active developer path, missing xcrun 错误 解决办法 图文教程’, ‘href’: ‘https://www.jiloc.com/44310.html’}
{‘title’: ‘WordPress 采集插件 WP-JPost 在Windows/Linux 系统环境下安装curl 采集 带ssl的https网站 图文教程’, ‘href’: ‘https://www.jiloc.com/43750.html’}{‘title’: ‘WordPress 用户删除’, ‘href’: ‘https://www.jiloc.com/44052.html’}
{‘title’: ‘WordPress 重设密码’, ‘href’: ‘https://www.jiloc.com/43961.html’}
{‘title’: ‘WordPress PHP 获取当前固定链接格式构造方法 图文教程’, ‘href’: ‘https://www.jiloc.com/43806.html’}
{‘title’: ‘CentOS下安装Apache Bench进行网站压力测试 ab工具使用 图文教程’, ‘href’: ‘https://www.jiloc.com/43885.html’}
{‘title’: ‘WordPress 个人档案’, ‘href’: ‘https://www.jiloc.com/44046.html’}
{‘title’: ‘BandwagonHost搬瓦工VPS快速安装BT宝塔Web面板(适合所有Linux VPS)’, ‘href’: ‘https://www.jiloc.com/43928.html’}
{‘title’: ‘WordPress函数:add_theme_page()后台添加设置页面’, ‘href’: ‘https://www.jiloc.com/43842.html’}
{‘title’: ‘WordPress 自动采集插件 wp-jpost 采集网站 dmzj.com 规则示例’, ‘href’: ‘https://www.jiloc.com/43748.html’}
{‘title’: ‘WordPress函数:load_theme_textdomain()(载入本地化语言文件)’, ‘href’: ‘https://www.jiloc.com/43836.html’}
{‘title’: ‘WordPress 自动采集插件 wp-jpost 采集网站 vs.cm 规则示例’, ‘href’: ‘https://www.jiloc.com/43743.html’}{‘title’: ‘WordPress 自动采集插件 wp-jpost 采集网站 eastmoney.com 规则示例’, ‘href’: ‘https://www.jiloc.com/43802.html’}
{‘title’: ‘WordPress函数:wp_tag_cloud(标签云)详解和举例’, ‘href’: ‘https://www.jiloc.com/43834.html’}
{‘title’: ‘WordPress 使用 Cloudflare 免费SSL Flexible模式 导致重定向过多问题 解决方案’, ‘href’: ‘https://www.jiloc.com/43922.html’}{‘title’: ‘上海溯飏船舶物资有限公司’, ‘href’: ‘https://www.jiloc.com/43797.html’}
{‘title’: ‘WordPress 自动采集插件 wp-jpost 采集网站 zimuzu.tv 规则示例’, ‘href’: ‘https://www.jiloc.com/43733.html’}
{‘title’: ‘WordPress 自动采集插件 wp-jpost 采集网站 i4.cn 规则示例’, ‘href’: ‘https://www.jiloc.com/43738.html’}
time cost : 9.66 s
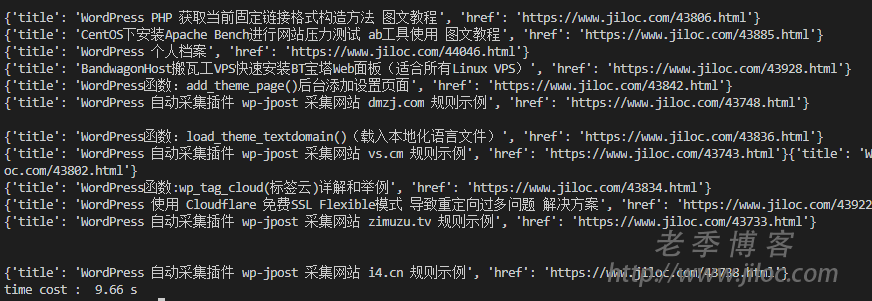
小结
不得不说python的采集速度是非常快的,总共就花了9.66秒。这个速度比PHP快乐N多倍。
发表回复